SpringMVC源码的分析
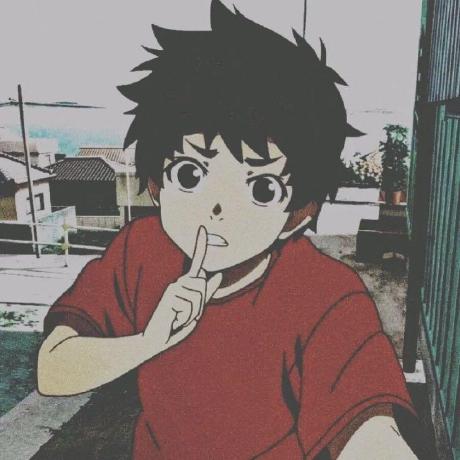
SpringMVC执行过程
流程图
Spring MVC 的四大组件:前端控制器(DispatcherServlet)、处理器映射器(HandlerMapping)、处理器适配器(HandlerAdapter)以及视图解析器(ViewResolver) 的角度来看一下 Spring MVC 对用户请求的处理过程,过程如下图所示:
其中,DispatcherServlet是前端控制器,它负责接收用户的请求,并调用其他组件来处理。HandlerMapping是处理器映射器,它负责根据请求的URL找到对应的Controller对象,并返回给DispatcherServlet。HandlerAdapter是处理器适配器,它负责根据Controller对象的类型,找到合适的适配器对象,并调用Controller对象的方法,返回ModelAndView对象。ModelAndView对象包含了模型数据和视图名称。ViewResolver是视图解析器,它负责根据视图名称找到对应的视图对象,并返回给DispatcherServlet。View是视图对象,它负责渲染模型数据,并返回响应给用户。
这样一来,springmvc就实现了一个基于MVC模式的web框架,将请求处理分成了不同的步骤和组件,提高了可扩展性和灵活性。
执行过程和组件介绍
- 用户向服务器发送请求,请求会先达到 SpringMVC 前端控制器 DispatcherServlet ;
- DispatcherServlet 根据该 URI,调用 HandlerMapping 获得该 Handler 配置的所有相关的对象(包括 Handler 对象以及 Handler 对象对应的拦截器),最后以 HandlerExecutionChain 执行链对象的形式返回
- DispatcherServlet 根据获得的 Handler,获取对应的 HandlerAdapter;
- 获取到 HandlerAdapter,将开始执行拦截器的 preHandler(…)方法;
- 提取 Request 中的模型数据,填充 Handler 入参,开始执行 Handler(Controller)方法,处理请求,在填充 Handler 的入参过程中,根据你的配置,Spring 将帮你做一些额外的工作:
- HttpMessageConveter:将请求消息(如 Json、xml 等数据)转换成一个对象,将对象转换为指定的类型信息
- 数据转换:对请求消息进行数据转换。如 String 转换成 Integer、Double 等
- 数据格式化:对请求消息进行数据格式化。如将字符串转换成格式化数字或格式化日期等
- 数据验证:验证数据的有效性(长度、格式等),验证结果存储到 BindingResult 或 Error 中
- Handler 方法执行完成后,向 DispatcherServlet 返回一个 ModelAndView 对象。
- 开始执行拦截器的 postHandle(…)方
- 根据返回的 ModelAndView(此时会判断是否存在异常:如果存在异常,则执行 HandlerExceptionResolver 进行异常处理)选择一个适合的 ViewResolver 进行视图解析,根据 Model 和 View,来渲染视图
- 渲染视图完毕执行拦截器的 afterCompletion(…)方法
- 将渲染结果返回给客户端,本次http请求完成
分析源码
准备工作
1. 创建Filter
1 |
|